SEC-Kit
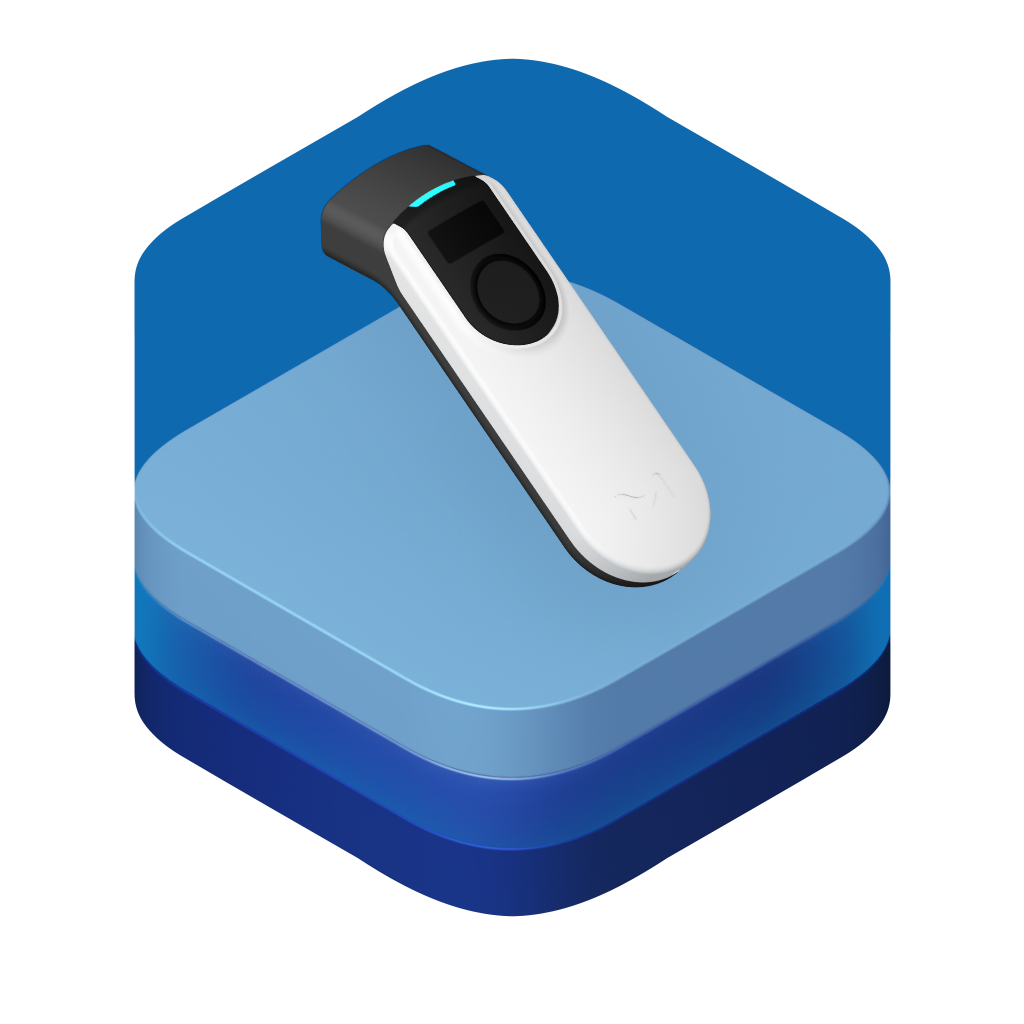
The M-Trust SEC-Kit allows you to integrate the SEC-Reader with your mobile applications. The SDK is available for iOS and Android.
Pre-requisites
Make sure you have flutter installed and have configured a Flutter project. You can find more information on how to set up a Flutter project in the official Flutter documentation.
Installation
Add the SEC-Kit and the BLE Strategy to your to your pubspec.yaml
file:
flutter pub add mtrust_sec_kit mtrust_urp_ble_strategy
For the pre-built UI to work you will need to also add the fonts to the pubspec.yaml
file:
flutter:
fonts:
- family: Lato
fonts:
- asset: packages/liquid_flutter/fonts/Lato-Regular.ttf
weight: 500
- asset: packages/liquid_flutter/fonts/Lato-Bold.ttf
weight: 800
- family: LiquidIcons
fonts:
- asset: packages/liquid_flutter/fonts/LiquidIcons.ttf
Permissions
For BLE to work, you need to add the respective permissions to the AndroidManifest.xml
and Info.plist
files.
<!-- Tell Google Play Store that your app uses Bluetooth LE
Set android:required="true" if bluetooth is necessary -->
<uses-feature android:name="android.hardware.bluetooth_le" android:required="false" />
<!-- New Bluetooth permissions in Android 12
https://developer.android.com/about/versions/12/features/bluetooth-permissions -->
<uses-permission android:name="android.permission.BLUETOOTH_SCAN" android:usesPermissionFlags="neverForLocation" />
<uses-permission android:name="android.permission.BLUETOOTH_CONNECT" />
<!-- legacy for Android 11 or lower -->
<uses-permission android:name="android.permission.BLUETOOTH" android:maxSdkVersion="30" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" android:maxSdkVersion="30" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" android:maxSdkVersion="30"/>
<!-- legacy for Android 9 or lower -->
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" android:maxSdkVersion="28" />
To avoid errors in release builds, please add the following line into your project/android/app/proguard-rules.pro file.
-keep class com.mtrust.sec_kit.** { *; }
iOS: Add the required permission to your ios/Runner/Info.plist file
<key>NSBluetoothAlwaysUsageDescription</key>
<string>This app always needs Bluetooth to function</string>
<key>NSBluetoothPeripheralUsageDescription</key>
<string>This app needs Bluetooth Peripheral to function</string>
<key>NSLocationAlwaysAndWhenInUseUsageDescription</key>
<string>This app always needs location and when in use to function</string>
<key>NSLocationAlwaysUsageDescription</key>
<string>This app always needs location to function</string>
<key>NSLocationWhenInUseUsageDescription</key>
<string>This app needs location when in use to function</string>
Usage
The most straight forward way to integrate the SEC Reader is using the SECSheet
widget. This widget shows a pre-built sheet at the bottom of the screen that allows the user to connect to the reader and start scanning.
The pre-built UI requires a theme to be set up and a a portal to be created.
Provide the theme somewhere at the top of your widget tree:
LdThemeProvider(
child: ...)
Wrap your Scaffold
with the LdPortal
widget:
LdPortal(
child: Scaffold(
...
)
)
In your widget, create a URPBleStrategy
instance:
late final _bleStrategy = UrpBleStrategy();
you are responsible for disposing the strategy when it is no longer needed:
void dispose() {
_bleStrategy.dispose();
super.dispose();
}
Then you can use the SecSheet
widget:
SecSheet(
strategy: _bleStrategy,
payload: "<my payload>",
onVerificationDone: (measurement) {
// handle the verification result
},
onVerificationFailed: () {
// handle the verification failure
},
builder: (context, openSheet) {
// You can use the openSheet function to open the sheet
return ElevatedButton(
onPressed: openSheet,
child: const Text("Open SEC Sheet"),
);
},
),
The strategy
is an instance of UrpBleStrategy
and the payload
is a string that will be sent to the reader and returned in the signed measurement result.
The onVerificationDone
and onVerificationFailed
callbacks are called when the verification is done or failed.